Content Security Policies with Angular
18-09-2024
For existing projects my task was to improve the security and add CSP-Headers (Among other things). When I added the headers to the servers response I got a lot of errors and the website was not rendering correctly anymore, because a lot of elements have been blocked. With this guide I want to help you to solve these issues and make your app more secure.
Read more »Conditional Guard with Angular
17-09-2024
I use CanDeactivate in one of my projects to prevent the user from leaving a form that has unsaved changes which is indicated by its dirty state. Whenever there are still unsaved changes a confirmation message is displayed. Unfortunately the message was also displayed in inappropriate cases e.g. when the user is logged out because his session expired. To prevent this I did some research and came up with pretty simple solution.
Read more »Google reCAPTCHA v3 with Angular
05-09-2024
Recently I had to implement a recaptcha in one of our projects. I was looking for an npm package, but even the popular ng-recaptcha package didn't support Angular version 18.
After checking the javascript code I came up with a simple solution, by building my own typescript service to wrap the javascript library from google.
Read more »After checking the javascript code I came up with a simple solution, by building my own typescript service to wrap the javascript library from google.
Dark mode with Angular Material
30-04-2024
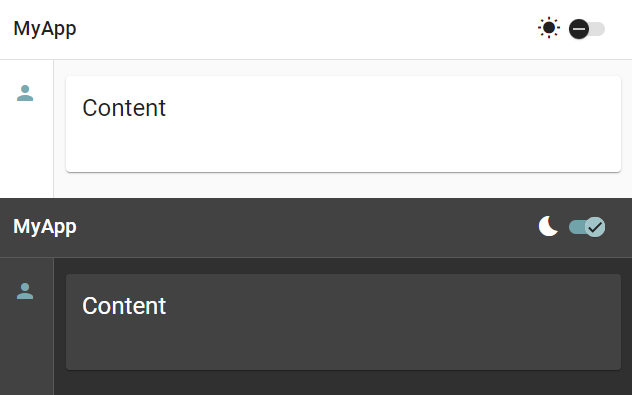
A dark mode can improve the accessibility of your website. In this article I want to show you how to implement a dark mode with Angular Material. This will include detection of the preferred mode of the browser and also a toggle button to switch between the modes. The last selection will be stored in the localstorage and can be restored the next time the website is loaded.
Read more »Dynamic localization with angular
24-04-2024
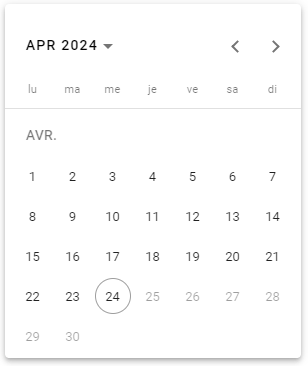
Recently we noticed that some parts of our UI don't get updated, when the language is changed. We are using dynamic translations with ngx-translate library so we can change the language inside the application at runtime and don't need to compile an application for each language, as it's intended by Angulars basic localization system. I did some research and was surprised, that it's still very complicated to achieve dynamic localization with Angular. I want to summarize the required steps here how to achieve dynamic translations, so you don't have to search through all the different sources.
Read more »Display unhandled client exceptions with Blazor
17-04-2024
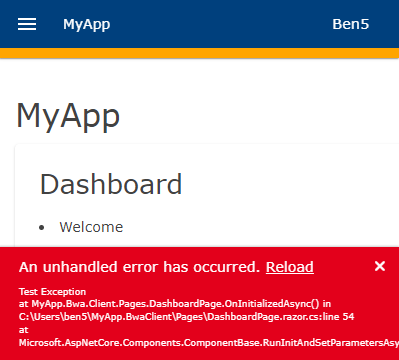
Unfortunately, the default error message for unhandled exceptions of Blazor doesn't provide any information of the exception. What I needed was to show these information to test user on a test system so it's easier to report errors.
An extension of the default blazor error message ("An unhandled error has occurred. Reload") which includes the exception message and stack trace would be ideal.
Read more »An extension of the default blazor error message ("An unhandled error has occurred. Reload") which includes the exception message and stack trace would be ideal.
Find unreferenced entities with Entity Framework Core
23-02-2024
One of my projects stores meta data of files in the database. The information is held in a FileEntity. Any other entity can then have a relation to this FileEntity.
The idea is, that files are uploaded first and are referenced afterwards. For example when a file for a photo album is uploaded, it will first create a FileEntity and then a PhotoAlbumEntity that contains a reference to the FileEntity.
The downside is that unreferenced files are using up space. So I had to find a way to find unreferenced file entities with Entity Framework Core.
Read more »Why you should always define column lengths
13-12-2023
One of our projects recently had severe performance issues which were caused by database queries. Eventually we figured out that the problem was the memory allocation caused by table columns which were all using NVARCHAR(MAX).
Read more »Access SOAP service with certificate and authentication
12-12-2023
Today I had a task to consume a SOAP endpoint from an external source. The documentation says that base authentication is required but also a client certificate has to be used to establish the SSL connection. I was trying for several hours to accomplish both, but it seemed that it was not that simple.
Read more »Let’s create a mobile game with Xamarin and OpenGL – Part 7 (State)
31-10-2023
You may have noticed in the last part, that whenever you leave the game, or even change the screen orientation, you will lose the current state of the game. In this part we are going to learn how we can keep the state of our running game.
Read more »Let’s create a mobile game with Xamarin and OpenGL – Part 6 (TicTacToe)
30-10-2023
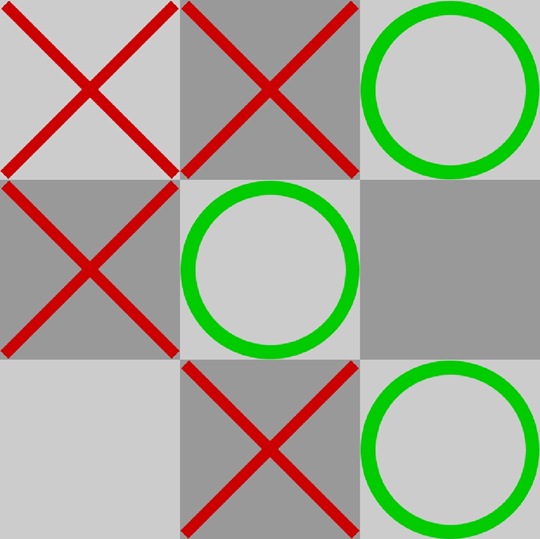
In this part we will finally create a fully playable game! TicTacToe is the perfect game to start with, because everybody knows it and it’s easy to implement.
Read more »Let’s create a mobile game with Xamarin and OpenGL – Part 5 (Scenes)
29-10-2023
In this part we are going to implement a scene. Scenes are helpful when you need to display different views in your game. Also scenes will make it possible to separate specific game code from the engine code. The first scene your game will show is usually a menu where the user can choose to start the game or enter the settings. Another scene might be the actual game where you render sprites that are controlled by user interaction.
Read more »Let’s create a mobile game with Xamarin and OpenGL – Part 4 (User input)
28-10-2023
In this part we are looking at important aspect of games, handling the user input. Without user interaction a game is not playable. Luckily it’s pretty simple to integrate user input in our project. In this article we will simply show or hide a sprite, whenever the screen is touched.
Read more »Let’s create a mobile game with Xamarin and OpenGL – Part 3 (Textures)
15-10-2023
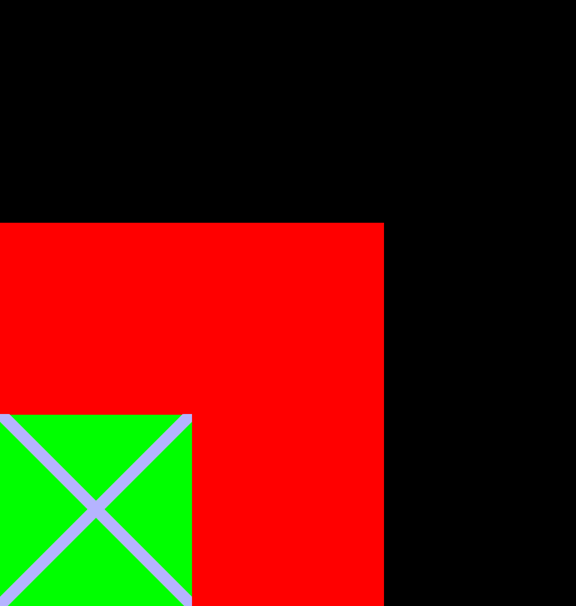
In this part we will continue with a more advanced shader which will allow us to draw rectangles with textures also known as sprites. Sprites are a main feature for simple 2D games and we can already achieve a lot by using them. You can use them for simple images, animations and also for rendering text.
Read more »Let’s create a mobile game with Xamarin and OpenGL – Part 2 (Shaders)
12-10-2023
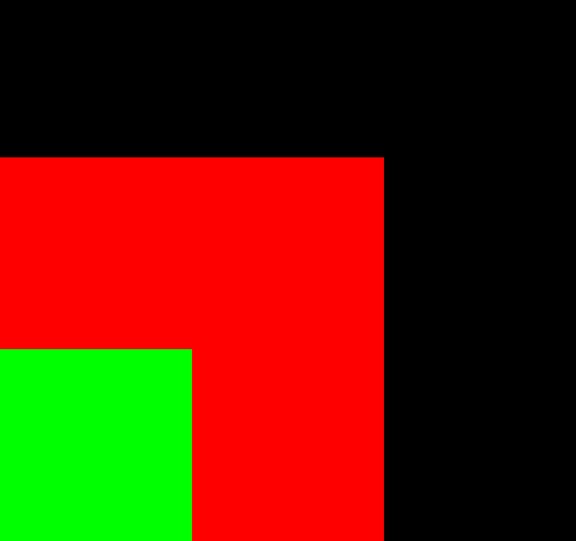
In this part I’m going to show you how you can use simple shaders to draw objects. We will start by drawing simple rectangles which are filled with a color.
Read more »Let’s create a mobile game with Xamarin and OpenGL – Part 1 (Setup)
11-10-2023
In this blog series I want to talk about a matter of heart. Since I was a child I always wanted to create my own computer game. I started with C++ and bought a book about how to create a game with DirectX. After a lot of time I managed to create my own games. But it was very complicated and took a lot of time. Four years ago I started developing a mobile game called “Mouse revenge”. Since I wanted it to become a mobile game I chose C# and Xamarin. In the beginning I was really struggling to find a good tutorial on how to create a platform independent game with Xamarin. So I tried a lot myself and finally found a structure that I could use to write only little platform dependent code and have most part of the game engine separated. After experiencing some bugs on specific devices I managed to have a stable version with zero crashes since months. I think it’s the right time to share my knowledge to everyone who wants to create his own mobile game with a good programming language.
Read more »Mixing Select() and Include()
10-10-2023
Last week I have been reviewing some code of one of our newer projects. I noticed that for some projections, also Include() was used:
Read more »Consecutive number per year with Entity Framework Core
09-10-2023
Recently a requirement for a project was that there has to be a registrations table with a column with the following format “2023_0001”. This format contains the year and a consecutive number. Each year, the number has to start with 1 again.
In this post I want to present some possible solutions for this problem and weigh up arguments against each other to find a reliable solution.
Read more »MatDialog changes in Angular Material 15
08-10-2023
Since Angular Material 15 the MatDialogs look quite different than they used to. Especially the font sizes are different. The CSS has changed:
Read more »Expression expanding with Entity Framework Core
07-10-2023
One of the greatest features I have discovered in the last few years is expression expanding for entity framework core. Usually when you create a projection with linq you will use the Select() method and either use an anonymous type or map the values you need from the database to a class. These projections are often faster because they don’t have to retrieve all the columns from the database table.
What I always missed was to be able to reuse a projection inside another projection. This can be helpful if you have expandable structures you want to provide to your API.
Read more »BadParcelableException in Android 13
06-10-2023
When Android 13 was introduced, I noticed in my Google Play Console that a lot of users are getting new exceptions almost daily.
Mostly it were “java.lang.NoSuchFieldException” or “android.os.BadParcelableException” exceptions.
Read more »First() vs. Single() for unique columns
05-10-2023
Recently during work, my team came up with the question how to query database entries that have a unique key. Should we use First or Single?
We already knew that First will make a database call where it will limit the result to 1 row. Single will limit the result to 2 rows, to determine if there is more than one result and if so, can throw an exception.
Read more »